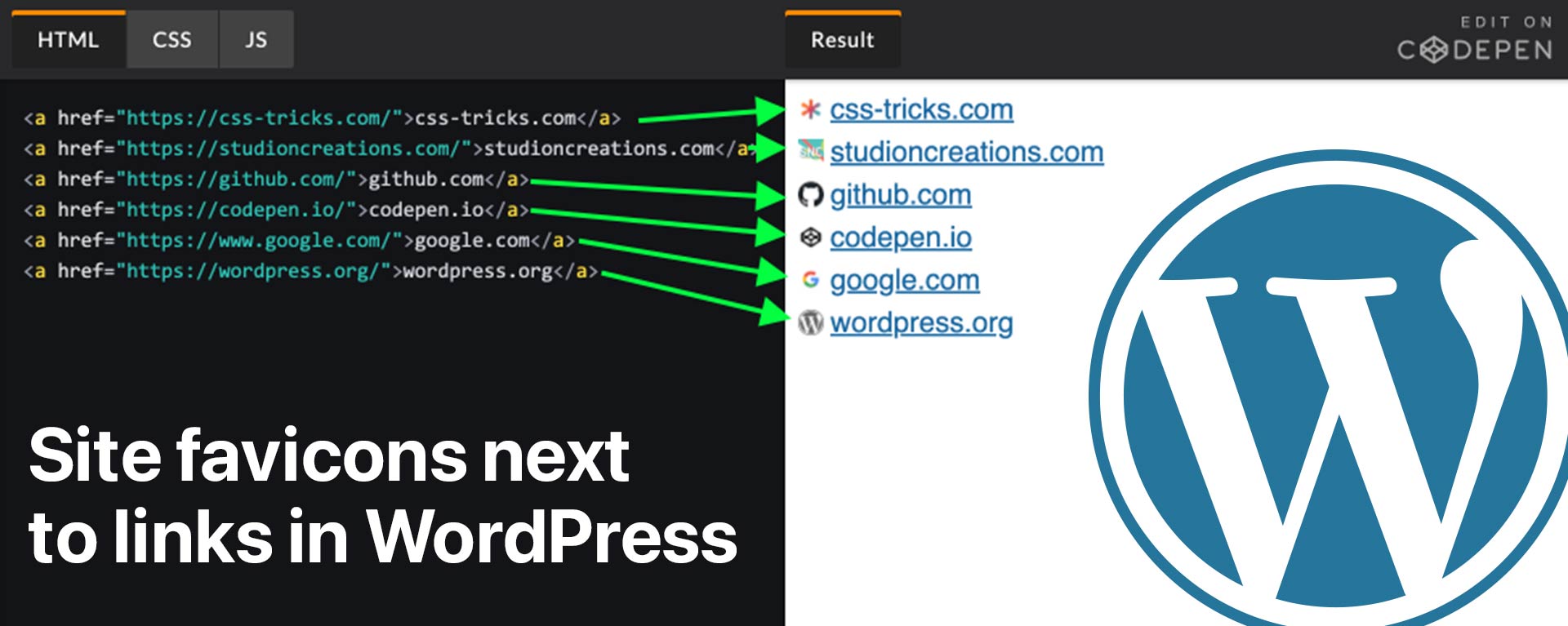
How to display favicons next to external links in WordPress
I stumbled upon this concept on CSS-Tricks the other day and thought it was the coolest idea. There were some issues that I quickly ran into though as I attempted to implement it on my WordPress site, but eventually I got it figured out.
If you’d like more background on the technique, you can check out Chris Coyier’s original article on css-tricks.com. For the most part, his tutorial works out-of-the-box, but it’s the usage of jQuery with the WordPress CMS that can cause some issues. Here’s the solution to displaying a favicon next to external links on your WordPress website.
First, the code snippet
I’ll cut right to the code. Chris goes into more detail about how it works, but for this article, we’re just concerned with getting it to function within WordPress.
$('a:not([href^="https://your-website.com/"]):not([href^="#"]):not([href^="/"])').each(function() { $(this).css({ background: "url(https://www.google.com/s2/favicons?domain=" + this.hostname + ") left center no-repeat", "padding-left": "20px" }); });
That first line of the code says not to add favicons to any link (<a>
) that begins with your domain (:not([href^="https://your-website.com/"])
), is not an in-page anchor link (:not([href^="#"])
), and is not an absolute path from your site root (:not([href^="/"])
). Be sure to change your-website.com
with your actual domain.
The key change
Normally we could paste that right into our markup, but due to compatibility proofing, WordPress doesn’t make use of jQuery’s $
symbol. To address this issue, swap $
with jQuery
.
jQuery('a:not([href^="https://your-website.com/"]):not([href^="#"]):not([href^="/"])').each(function() { jQuery(this).css({ background: "url(https://www.google.com/s2/favicons?domain=" + this.hostname + ") left center no-repeat", "padding-left": "20px" }); });
Adding it to a WordPress theme
If you’re working with a static site, you would just paste the entire script into your page markup. WordPress generates dynamic pages though, so we need to use our theme’s functions.php
to add the code snippet upon page load.
Here is exactly what to insert into your functions.php
. You can paste it at the end of the file.
/* Show favicons next to external links */ add_action('wp_footer', 'external_link_favicons_js'); function external_link_favicons_js() { ?> <script> jQuery('a:not([href^="https://your-website.com/"]):not([href^="#"]):not([href^="/"])').each(function() { jQuery(this).css({ background: "url(https://www.google.com/s2/favicons?domain=" + this.hostname + ") left center no-repeat", "padding-left": "20px" }); }); </script> <?php }
An important tweak
There’s one issue with just using the snippet above as-is though. Whenever you have an image in a post that links to an external source, that image will also have a favicon next to it, and we don’t want that.
I use Jetpack CDN for all of my images, and in this screenshot you can see the Jetpack favicon next to my photo.
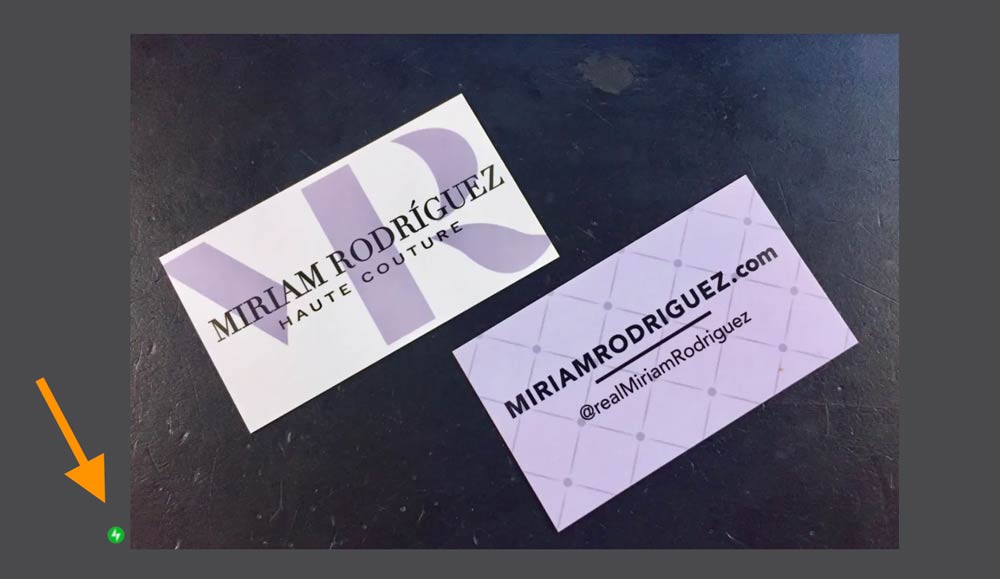
When inserting an image into a Post using Gutenberg, WordPress automatically places the img
within a figure
. To remove the favicon before your image, add this CSS snippet into your functions.php
, beneath the first snippet above.
/* Don't show favicons for <a><img> inside <figure> */ add_action('wp_head', 'external_link_favicons_css'); function external_link_favicons_css() { echo '<style>figure > a {background: none !important;padding-left: 0 !important;}</style>'; }
The finalized code
Here’s the full code snippet. Put it into your WordPress theme’s functions.php
(preferably at the bottom) in order to display a favicon next to all external links that aren’t images.
/* Show favicons next to external links within a Post*/ add_action('wp_footer', 'external_link_favicons_js'); function external_link_favicons_js() { ?> <script> jQuery('a:not([href^="https://your-website.com/"]):not([href^="#"]):not([href^="/"])').each(function() { jQuery(this).css({ background: "url(https://www.google.com/s2/favicons?domain=" + this.hostname + ") left center no-repeat", "padding-left": "20px" }); }); </script> <?php } /* Don't show favicons for <a><img> inside <figure> */ add_action('wp_head', 'external_link_favicons_css'); function external_link_favicons_css() { echo '<style>figure > a {background: none !important;padding-left: 0 !important;}</style>'; }
Thanks for reading!